2.Python中组的概念
2.1 列表(list: 可变的)
初识列表:1
2
3
4
5
6
7
8
9
10>>> type([1,2,3])
<class 'list'>
>>> type([1,2,'test',True,7j])
<class 'list'>
>>> type([[3,'st'],[9,False,'be'],[5]]) #嵌套列表 类似二维数组等概念,但元素的类似可以是任意一种
<class 'list'>
>>> type([(3,'st'),{1,1,'sdo'},[9,False,'be'],[5]]) # 十分灵活
<class 'list'>
>>>
常用基本的访问列表方法(同str很像):列表之间只能相加,列表与数字可以相乘1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23>>> ['坚韧','致命打击','勇气','审判','德玛西亚大宝剑'][1] # 不使用冒号,直接访问 得到的是一个值
'致命打击'
>>> ['坚韧','致命打击','勇气','审判','德玛西亚大宝剑'][4:5] # 使用冒号,结果依旧是个列表
['德玛西亚大宝剑']
>>> ['坚韧','致命打击','勇气','审判','德玛西亚大宝剑'][-1:]
['德玛西亚大宝剑']
>>>
>>> ['引燃','闪现'] + ['治疗','传送'] # 列表之间可以相加操作
['引燃', '闪现', '治疗', '传送']
>>> ['引燃','闪现'] * 2 # 列表可与数字相乘
['引燃', '闪现', '引燃', '闪现']
>>> ['引燃','闪现'] * ['引燃','闪现'] # 列表之间不能相乘
Traceback (most recent call last):
File "<pyshell#36>", line 1, in <module>
['引燃','闪现'] * ['引燃','闪现']
TypeError: can't multiply sequence by non-int of type 'list'
>>> ['引燃','闪现'] - ['引燃','闪现'] # 列表之间不能相减
Traceback (most recent call last):
File "<pyshell#37>", line 1, in <module>
['引燃','闪现'] - ['引燃','闪现']
TypeError: unsupported operand type(s) for -: 'list' and 'list'
>>>
2.2 元组(Tuple: 不可变的)
元组(Tuple)很多性质和列表(list)一样,但有些地方不一样(后续会总结其不同的地方).1
2
3
4
5
6
7
8
9
10
11
12
13
14
15>>> type((1,2,3))
<class 'tuple'>
>>> (1,'asd',True,('t',2))
(1, 'asd', True, ('t', 2))
>>> (1, 'asd', True, ('t', 2))[3]
('t', 2)
>>> (1, 'asd', True, ('t', 2))[0:2]
(1, 'asd')
>>> (1, 'asd', True, ('t', 2))[-1:]
(('t', 2),)
>>> (1, 'asd', True, ('t', 2))+(5,5)
(1, 'asd', True, ('t', 2), 5, 5)
>>> (1,2)*2
(1, 2, 1, 2)
>>>
另外还有一个现象:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16>>> type((1)) # 因为在Python中()会被默认为是在做数学运算,所以会被当做int类型
<class 'int'> # 明明应该是元组(Tuple)的好嘛!
>>> type(('s'))
<class 'str'> # 明明应该是元组(Tuple)的好嘛!
>>> type(('s',))
<class 'tuple'> # 只是加了逗号,就成tuple了 =_=
type((1,))
<class 'tuple'>
>>> (1+2)*2
6
>>> (1) # 结果是整数1
1
>>> (1,) # 结果是tuple元组
(1,)
>>> type(( )) # 空元组tuple
<class 'tuple'>
2.3 序列小结
小结一下:number(int,float,bool)、str、list、tuple,
其中str,list,tuple都是序列,拥有相同的特性:
a.里边的每个元素都有一个序列号,是有顺序的;
b.可以进行切片操作;1
2
3
4
5
6
7
8
9
10
11
12>>> (1,2,3,4,5,6)[-1:]
(6,)
>>> (1,2,3,4,5,6)[5:6]
(6,)
>> (1,2,3,4,5,6)[0:4:2] # 就叫‘三元切片’吧..
(1, 3)
>>> (1,2,3,4,5,6)[0:4:3]
(1, 4)
>>> (1,2,3,4,5,6)[0:4:4]
(1,)
>>>
c. 可以进行 +、* 操作;
d.判断某个元素是否在序列里面,返回结果为bool类型;1
2
3
4
5
6
7>>> 1 in (1,23,5)
True
>>> 's' not in ['q',2,4]
True
>>> '1d' in 'sfk1d'
True
>>>
e. 一些通用操作:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16>>> len((1,2,3))
3
>>> max('abcde')
'e'
>>> min([1,4,6])
1
>>> min([1,3,'5']) # 不同类型之间无法比较!
Traceback (most recent call last):
File "<pyshell#5>", line 1, in <module>
min([1,3,'5'])
TypeError: '<' not supported between instances of 'str' and 'int'
>>> ord('d') # 将字母d的ASCII求出来
100
>>> ord(' ')
32
>>>
2.4 集合(set)
集合特点:
a. 无序且元素不可重复。1
2
3
4
5
6
7
8>>> {1,3,5}[0] # 无法根据下标进行取值操作
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
{1,3,5}[0]
TypeError: 'set' object does not support indexing
>>> {1,5,7,6,5,1} # 因为元素是无序的,所以不可重复
{1, 5, 6, 7}
>>>
b.可对集合进行的一般操作:1
2
3
4
5
6
7
8
9>>> len({1,2,'d',True}) # 求长度
3
>>> max({1,2,5}) # 最大最小值等
5
>>> 1 in {1,3,7}
True
>>> 1 not in {1,6,8}
False
>>>
c. 集合的一些特色操作1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18>>> {1,3,5,7} - {1,3,6} # 求两个集合的差集 -
{5, 7}
>>> {1,3,5,7} & {1,3,6} # 求两个集合的交集 &
{1, 3}
>>> {1,3,5,7} |{1,3,6} #并集 |
{1, 3, 5, 6, 7}
>>> type({}) # 空集合是如何定义的呢?
<class 'dict'>
>>> type(set()) #空集合的真正定义方法是这样的,同tuple很像
<class 'set'>
>>> type(())
<class 'tuple'>
>>> len(set())
0
>>> len(())
0
>>>
2.5 字典(dict)
字典也是一种集合,是无序的。1
2
3
4
5
6
7
8
9
10
11
12
13
14
15>>> type({1:'q',2:'a',3:True})
<class 'dict'>
>>> {'Q':'致命打击','W':'勇气','E':'审判'}[1] # 不能通过下标访问,说明是无序的
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
{'Q':'致命打击','W':'勇气','E':'审判'}[1]
KeyError: 1
>>> {'Q':'致命打击','W':'勇气','E':'审判'}['W']
'勇气'
>>> {'Q':'致命打击','Q':'沉默','W':'勇气','E':'审判'}['Q']
'沉默'
>>> {'Q':'致命打击','Q':'沉默','W':'勇气','E':'审判'} # 虽然没报错 但是key不要出现相同的
{'Q': '沉默', 'W': '勇气', 'E': '审判'}
>>> {1:'致命打击','1':'沉默','W':'勇气','E':'审判'}[1]
'致命打击'
dict的value几乎可以是Python中的任意一种类型,但是key必须是一种不可变的类型!
下面的例子也说明:元组tuple是不可变的,list列表是可变的。1
2
3
4
5
6
7
8
9
10>>> {[1,2]:'致命打击','Q':'沉默','W':'勇气','E':'审判'}
Traceback (most recent call last):
File "<pyshell#6>", line 1, in <module>
{[1,2]:'致命打击','Q':'沉默','W':'勇气','E':'审判'}
TypeError: unhashable type: 'list'
>>> {(1,2):'致命打击','Q':'沉默','W':'勇气','E':'审判'}
{(1, 2): '致命打击', 'Q': '沉默', 'W': '勇气', 'E': '审判'}
>>> type({}) #空字典的表示方法!
<class 'dict'>
Python基本数据类型总结:
如图,其中str及tuple都是不可变的,lists是可变的,不能用来做字典的key。
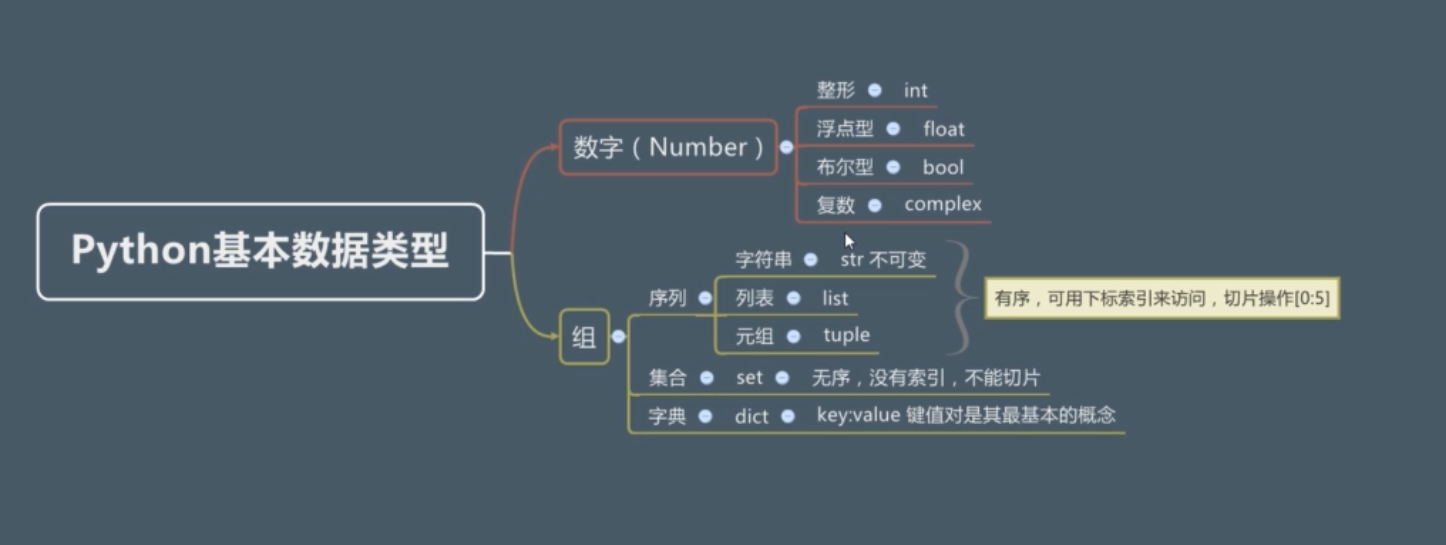