(基于IDLE的演示)
1.Python的基本类型1–Number
1.1 数字: 整形与浮点型、布尔与复数
Python中数字(Number)分为: (int)整形、(float)浮点型、(bool)布尔类型、(complex)复数。
int 与 float :1
2
3
4
5
6
7
8
9
10
11>>> type(1)
<class 'int'>
>>> type(1.1)
<class 'float'>
>>> type(1*2)
<class 'int'>
>>> type(2/2)
<class 'float'>
>>> type(2//2) #转义字符 即让两个整除的整数的结果变为整形
<class 'int'>
>>>
bool : True、False 首字母一定要大写!
1 | >>> type(True) |
在Python中为什么bool属于数字这一基本类型呢?1
2
3
4
5
6
7
8
9
10
11
12>>> int(False)
0
>>> int(True)
1
>>> bool(1)
True
>>> bool(0)
False
>>> bool(0b01) #二进制的1
True
>>> bool(0b0) #二进制的0
False
其他哪些数字或者字符也可以表示True或者False呢?1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18>> bool(2)
True
>>> bool(-1)
True
>>> bool(1.1)
True
>>> bool( ) # 空格表示False
False
>>> bool('') # 空字符串也表示False
False
>>> bool(' ') # 带有空格的字符串表示True
True
>>> bool([1,2,3]) # 非空列表(同理非空列表'{1,2,3}')表示True
True
>>> bool([]) # 空列表表示False
False
>>> bool(None) # None也表示False
False
1.2 字符串 str
表示方法: 单引号、双引号、三引号。
三种表示方法都可以,这样设计只是为了嵌套表达方便以及方便换行。
a.方便嵌套表达:1
2
3
4
5
6
7
8
9
10>>> type('hi nice')
<class 'str'>
>>> type("cat tom")
<class 'str'>
>>> type('''dog tony''')
<class 'str'>
>>> print("let's go")
let's go
>>> print('let\'s go') # '\' 转义字符
let's go
b.方便换行表达:1
2
3
4
5
6
7
8
9>>> '''first line
second line
third line'''
'first line\nsecond line\nthird line'
>>> """first line
second line
third line"""
'first line\nsecond line\nthird line'
>>>
反过来想输出多行。则需借助print函数实现:1
2
3
4
5
6
7
8
9
10
11
12>>> print("""hi Tom\nhi Jim\nhi Mary""")
hi Tom
hi Jim
hi Mary
>>> print('''hi Tom\nhi Jim\nhi Mary''')
hi Tom
hi Jim
hi Mary
>>> print('hi Tom\nhi Jim\nhi Mary')
hi Tom
hi Jim
hi Mary
再补充一种单引号换行方法:1
2
3
4
5
6
7>>> 'hi #直接按Enter换行会报错
SyntaxError: EOL while scanning string literal
>>> 'hi \ # 末尾加一个 \ 即可实现单引号换行
tom'
'hi tom'
>>>
1.3 转义字符及原始字符串
转义字符串即一些特殊字符,大概分为两类:
a. 无法“看见”的字符,比如 换行符(\n),横向制表符(\t),回车符(\r)
b. 与语言本身语法有冲突的字符,比如 单引号(\’)
更多的转义字符可网上查询。1
2
3
4
5
6
7
8
9
10
11
12>>> print('hi \n tom')
hi
tom
>>> print('hi \\n tom')
hi \n tom
>>> 'let\'s go'
"let's go"
>>> print('C:\\utils\\javaTools') # 通过转义字符输入目录
C:\utils\javaTools
>>>
原始字符串 r1
2
3>>> print(r'C:\utils\javaTools') # 通过原始字符串 r(也可大写R) 可以让 \ 原始输出
C:\utils\javaTools
>>>
1.4 字符串的相关运算
a.简单的 +、* 及基本的取值操作:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19>>> 'hi' + ' Tom'
'hi Tom'
>>> 'hi '*3
'hi hi hi '
>>> "nice to meet"[2]
'c'
>>> "nice to meet"[-1]
't'
>>> "hi nice !"[0:2]
'hi'
>>> "hi nice !"[0:-2]
'hi nice'
>>> "hello word"[6:10] # 大于字符串的最大长度也无所谓,这里10写20也可以
'word'
>>> "hello word"[6:] # 这里的冒号(空缺)表示从第六位到最后
'word'
>>> "dguhioefoy89y weg python"[-6:] # 字符串较长时如果只取最后几个字符 这种操作高效
'python'
python基本数据类型导图
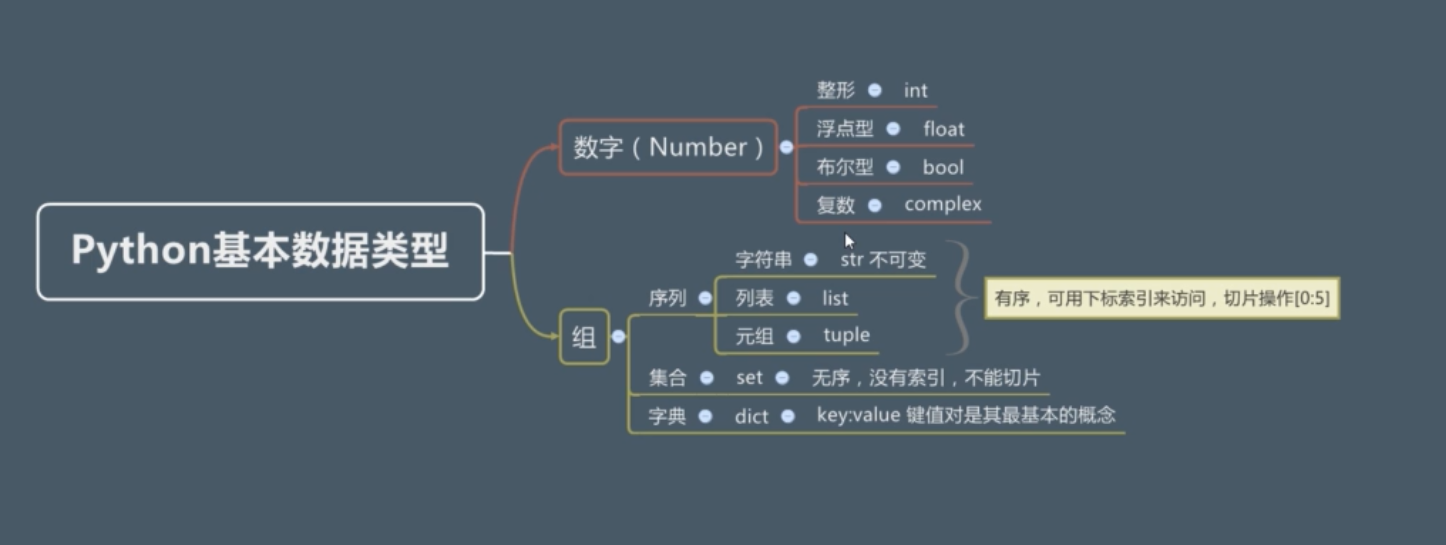